
- Python 3 Syntax Cheat Sheet
- Python Basic Syntax Pdf
- Python Syntax Guide
- Python Commands
Resources for the second edition are here. I'd love to know what you think about Python Crash Course; please consider taking a brief survey. If you'd like to know when additional resources are available, you can sign up for email notifications here.

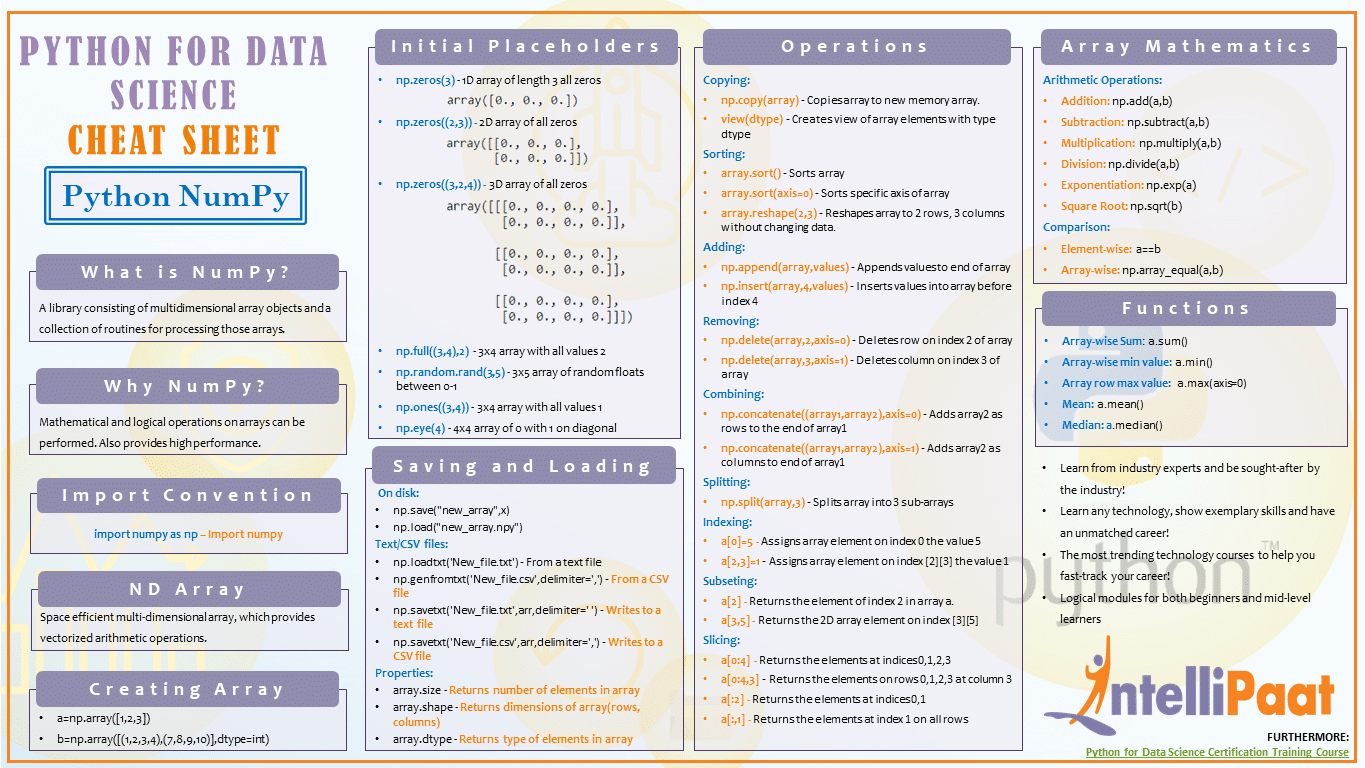
Cheat sheets can be really helpful when you’re trying a set of exercises related to a specific topic, or working on a project. Because you can only fit so much information on a single sheet of paper, most cheat sheets are a simple listing of syntax rules. This set of cheat sheets aims to remind you of syntax rules, but also remind you of important concepts as well. You can click here and download all of the original cheat sheets in a single document. Autocad for mac full crack.
Python 3 Syntax Cheat Sheet
There are 2 kinds of loops used in Python - the for loop and the while loop. For loops are traditionally used when you have a piece of code which you want to repeat n number of times. Macos catalina installer dmg. They are also commonly used to loop or iterate over lists. 1 colors = 'red', 'green', 'blue' 2 colors 3 'red', 'green', 'blue' 4 for color in colors.
- Python has integers and floats. Integers are simply whole numbers, like 314, 500, and 716. Floats, meanwhile, are fractional numbers like 3.14, 2.867, 76.88887. You can use the type method to check the value of an object. type(3) type(3.14) pi = 3.14 type(pi) Python Cheat Sheet 1. Primitives Numbers.
- Read and Write to CSV. pd.readcsv('file.csv', header=None, nrows=5).
- Syntax: $ ^formatspec $% numericexp-formatspec’syntax:’’% width.precision-type-x width$(optional):aligninnumberofcolums specified;negativetoleft Oalign, precede$with 0tozero Ofill-x precision$(optional):showspecifieddigitsof precisionforfloa ts;$6isdefault-x type (required):d(decimal$int),f(float),s (string),e(float t$exponential$notation).
- Welcome to Python Cheatsheet! ☕️ Anyone can forget how to make character classes for a regex, slice a list or do a for loop. This cheat sheet tries to provide a basic reference for beginner and advanced developers, lower the entry barrier for newcomers and help veterans refresh the old tricks.
An updated version of these sheets is also available through Leanpub and Gumroad. The updated version includes a sheet that focuses on Git basics, a printer-friendly b&w version of each sheet, and each sheet as a separate document. The updated versions are available at no cost on both platforms.
Individual Sheet Descriptions
- Beginner’s Python Cheat Sheet
- Provides an overview of the basics of Python including variables, lists, dictionaries, functions, classes, and more.
- Beginner’s Python Cheat Sheet - Lists
- Focuses on lists: how to build and modify a list, access elements from a list, and loop through the values in a list. Also covers numerical lists, list comprehensions, tuples, and more.
- Beginner’s Python Cheat Sheet - Dictionaries
- Focuses on dictionaries: how to build and modify a dictionary, access the information in a dictionary, and loop through dictionaries in a variety of ways. Includes sections on nesting lists and dictionaries, using an OrderedDict and more.
- Beginner’s Python Cheat Sheet - If Statements and While Loops
- Focuses on if statements and while loops: how to write conditional tests with strings and numerical data, how to write simple and complex if statements, and how to accept user input. Also covers a variety of approaches to using while loops.
- Beginner’s Python Cheat Sheet - Functions
- Focuses on functions: how to define a function and how to pass information to a function. Covers positional and keyword arguments, return values, passing lists, using modules, and more.
- Beginner’s Python Cheat Sheet - Classes
- Focuses on classes: how to define and use a class. Covers attributes and methods, inheritance and importing, and more.
- Beginner’s Python Cheat Sheet - Files and Exceptions
- Focuses on working with files, and using exceptions to handle errors that might arise as your programs run. Covers reading and writing to files, try-except-else blocks, and storing data using the json module.
- Beginner’s Python Cheat Sheet - Testing Your Code
- Focuses on unit tests and test cases. How to test a function, and how to test a class.
- Beginner’s Python Cheat Sheet - Pygame
- Focuses on creating games with Pygame. Creating a game window, rect objects, images, responding to keyboard and mouse input, groups, detecting collisions between game elements, and rendering text.
- Beginner’s Python Cheat Sheet - matplotlib
- Focuses on creating visualizations with matplotlib. Making line graphs and scatter plots, customizing plots, making multiple plots, and working with time-based data.
- Beginner’s Python Cheat Sheet - Pygal
- Focuses on creating visualizations with Pygal. Making line graphs, scatter plots, and bar graphs, styling plots, making multiple plots, and working with global datasets.
- Beginner’s Python Cheat Sheet - Django
- Focuses on creating web apps with Django. Installing Django and starting a project, working with models, building a home page, using templates, using data, and making user accounts.
Available from No Starch Press and Amazon.
Basics
Print a number | print(123) |
Print a string | print('test') |
Adding numbers | print(1+2) |
Variable assignment | number = 123 |
Print a variable | print(number) |
Function call | x = min(1, 2) |
Comment | # a comment |
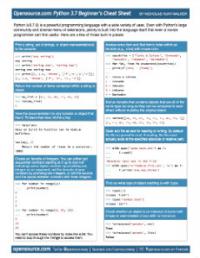
Types
Integer | 42 |
String | 'a string' |
List | [1, 2, 3] |
Tuple | (1, 2, 3) |
Boolean | True |
Useful functions
Write to the screen | print('hi') |
Calculate length | len('test') |
Minimum of numbers | min(1, 2) |
Maximum of numbers | max(1, 2) |
Cast to integer | int('123') |
Cast to string | str(123) |
Cast to boolean | bool(1) |
Range of numbers | range(5, 10) |
Other syntax
Return a value | return 123 |
Indexing | 'test'[0] |
Slicing | 'test'[1:3] |
Continue to next loop iteration | continue |
Exit the loop | break |
List append | numbers = numbers + [4] |
List append (with method call) | numbers.append(4) |
List item extraction | value = numbers[0] |
List item assignment | numbers[0] = 123 |
Terminology
syntax | the arrangement of letters and symbols in code |
program | a series of instructions for the computer |
print | write text to the screen |
string | a sequence of letters surrounded by quotes |
variable | a storage space for values |
value | examples: a string, an integer, a boolean |
assignment | using = to put a value into a variable |
function | a machine you put values into and values come out |
call (a function) | to run the code of the function |
argument | the input to a function call |
parameter | the input to a function definition |
return value | the value that is sent out of a function |
conditional | an instruction that's only run if a condition holds |
loop | a way to repeatedly run instructions |
list | a type of value that holds other values |
tuple | like a list, but cannot be changed |
indexing | extracting one element at a certain position |
slicing | extracting some elements in a row |
dictionary | a mapping from keys to values |
Reminders
- Strings and lists are indexed starting at 0, not 1
- Print and return are not the same concept
- The return keyword is only valid inside functions
- Strings must be surrounded by quotes
- You cannot put spaces in variable or function names
- You cannot add strings and integers without casting
- Consistent indentation matters
- Use a colon when writing conditionals, function definitions, and loops
- Descriptive variable names help you understand your code better
Conditionals
Lists
Defining functions
Loops
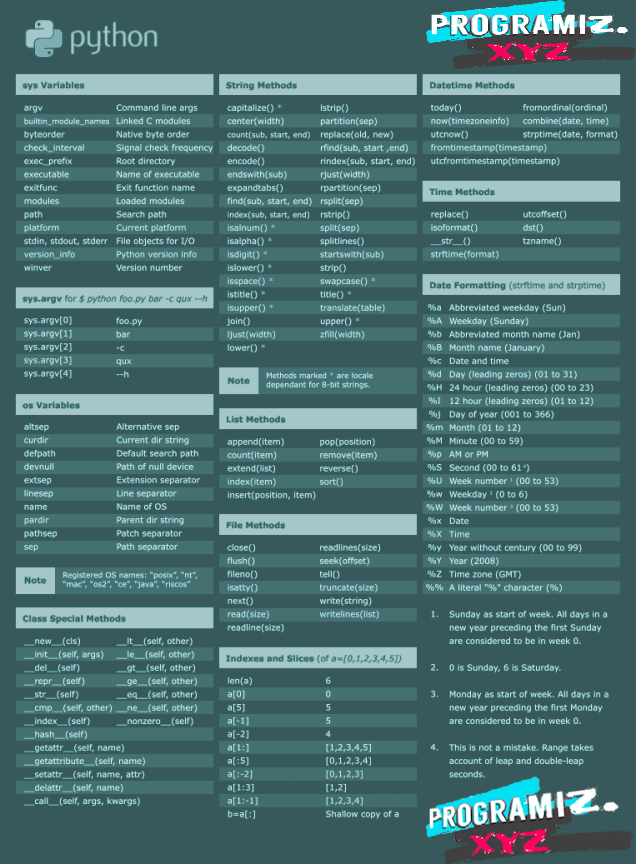
Dictionaries
Comparisons
Equals |
Not equals | != |
Less than | < |
Less than or equal | <= |
Greater than | > |
Python Basic Syntax Pdf
Useful methods
String to lowercase | 'xx'.lower() |
String to uppercase | 'xx'.upper() |
Split string by spaces | 'a b c'.split(' ') |
Remove whitespace around string | ' a string '.strip() |
Combine strings into one string | ' '.join(['a', 'b']) |
String starts with | 'xx'.startswith('x') |
String ends with | 'xx'.endswith('x') |
List count | [1, 2].count(2) |
List remove | [1, 2].remove(2) |
Dictionary keys | {1: 2}.keys() |
Dictionary values | {1: 2}.values() |
Dictionary key/value pairs | {1: 2}.items() |

Other neat bonus stuff
Python Syntax Guide
Zip lists | zip([1, 2], ['one', 'two']) |
Set | my_set = {1, 2, 3} |
Set intersection | {1, 2} & {2, 3} |
Set union | {1, 2} | {2, 3} |
Index of list element | [1, 2, 3].index(2) |
Sort a list | numbers.sort() |
Reverse a list | numbers.reverse() |
Sum of list | sum([1, 2, 3]) |
Numbering of list elements | for i, item in enumerate(items): |
Read a file line by line | for line in open('file.txt'): |
Read file contents | contents = open('file.txt').read() |
Random number between 1 and 10 | import random; x = random.randint(1, 10) |
List comprehensions | [x+1 for x in numbers] |
Check if any condition holds | any([True, False]) |
Check if all conditions hold | all([True, False]) |
Missing something?
Python Commands
Let us know here.